I wanted to try this option out so i built a HelloJersey application that you can download from here. I followed these steps for building my sample application
- First create a Dynamic Web APplication project called HelloJersey
- Download following .jar files and add it to the WEB-INF/lib folder of your web application
- asm-3.1.jar
- jersey-core-1.5.jar
- jersey-server-1.5.jar
- jsr311-api-1.1.1.jar
- Next create a HelloJerseyRESTService.java file like this
package com.webspherenotes.rest;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.MediaType;
@Path("/hellojersey")
public class HelloJerseyRESTService {
@GET
@Produces(MediaType.TEXT_HTML)
public String sayHello(@QueryParam("name") String name ){
return "Hello " + name;
}
}
The HelloJerseyRESTService only handles GET request and returns HTML markup
Next declare the Jersey servlet in the web.xml and create servlet mapping
<?xml version="1.0" encoding="UTF-8"?>
<web-app id="WebApp_ID" version="2.5"
xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<display-name>DynaCacheSample1</display-name>
<servlet>
<servlet-name>Jersey REST Service</servlet-name>
<servlet-class>com.sun.jersey.spi.container.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>com.sun.jersey.config.property.packages</param-name>
<param-value>com.webspherenotes.rest</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>Jersey REST Service</servlet-name>
<url-pattern>/rest/*</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
</web-app>
After you deploy the application in container you should see that when the server is starting it will scan all the resource and in our case it should find onlycom.webspherenotes.rest.HelloJerseyRESTService
[10/16/11 17:17:47:250 PDT] 0000000e ApplicationMg I WSVR0228I: User initiated module stop operation request completed on Module, HelloJersey.war, of application, DynaCacheSample1EAR
[10/16/11 17:17:49:932 PDT] 0000000e ApplicationMg I WSVR0225I: User initiated module start operation requested on Module, HelloJersey.war, of application, DynaCacheSample1EAR
[10/16/11 17:17:50:466 PDT] 0000000e webapp I com.ibm.ws.webcontainer.webapp.WebGroupImpl WebGroup SRVE0169I: Loading Web Module: HelloJersey.
[10/16/11 17:17:50:521 PDT] 0000000e WASSessionCor I SessionContextRegistry getSessionContext SESN0176I: Will create a new session context for application key default_host/HelloJersey
[10/16/11 17:17:50:602 PDT] 0000000e PackagesResou I Scanning for root resource and provider classes in the packages:
com.webspherenotes.rest
[10/16/11 17:17:50:666 PDT] 0000000e ScanningResou I Root resource classes found:
class com.webspherenotes.rest.HelloJerseyRESTService
[10/16/11 17:17:50:667 PDT] 0000000e ScanningResou I No provider classes found.
[10/16/11 17:17:50:850 PDT] 0000000e WebApplicatio I Initiating Jersey application, version 'Jersey: 1.5 01/14/2011 12:36 PM'
[10/16/11 17:17:51:816 PDT] 0000000e servlet I com.ibm.ws.webcontainer.servlet.ServletWrapper init SRVE0242I: [DynaCacheSample1EAR] [/HelloJersey] [Jersey REST Service]: Initialization successful.
[10/16/11 17:17:51:817 PDT] 0000000e webcontainer I com.ibm.ws.wswebcontainer.VirtualHost addWebApplication SRVE0250I: Web Module HelloJersey has been bound to default_host[*:9080,*:80,*:9443,*:5060,*:5061,*:443].
[10/16/11 17:17:51:824 PDT] 0000000e ApplicationMg I WSVR0226I: User initiated module start operation request completed on Module, HelloJersey.war, of application, DynaCacheSample1EAR
[10/16/11 17:17:51:825 PDT] 0000000e AppBinaryProc I ADMA7021I: Distribution of application DynaCacheSample1EAR completed successfully.
You can test the service by either directly going to the
http://localhost:9080/HelloJersey/rest/hellojersey?name=sunil
URL or using the REST client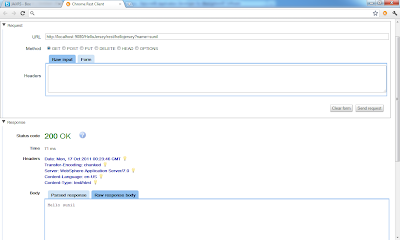
2 comments:
Thanks for info
Web Design Company in Bangalore
Website development in Bangalore
THANK YOU FOR THE INFORMATION
PLEASE VISIT US
Web Design Company in Bangalore
Post a Comment